メッセージを表示するScript2
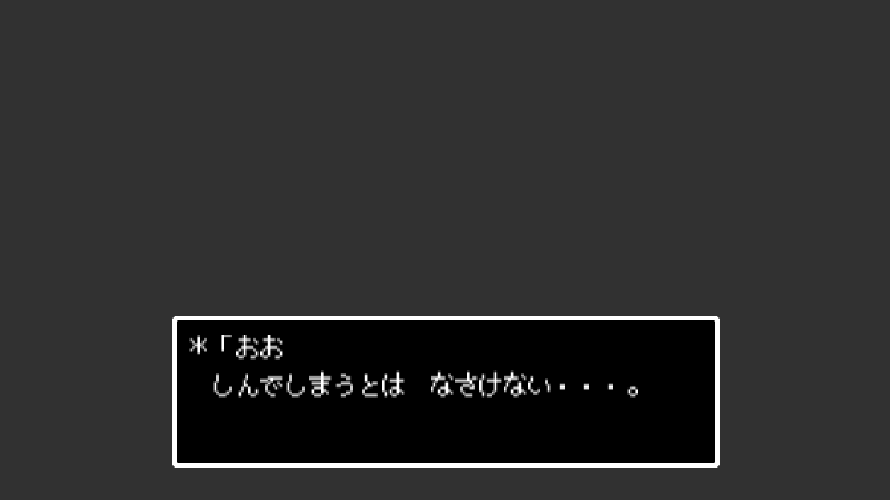
前回、メッセージを表示するScriptを掲載いたしました。このScriptでは古いメッセージを消去して新しいメッセージを表示していました。今回は、古いメッセージが上へ流れていくようにしてみました。
Script
作成したScriptはこちらです。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 |
using System.Collections; using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class CreateMessageV5 : MonoBehaviour { public GameObject preMessageWindow, preText, preSndMessage, preSndNextMessage, preMask; public Vector3 windowPosition; //メッセージウィンドウの位置 public Vector2 windowSize; //メッセージウィンドウの大きさ /* テキストはメッセージウィンドウと同じ位置に作られる */ public Vector2 textAreaSize; //テキスト領域の大きさ public int lineNumber = 3; //行数 public Vector3 textOffset; //位置調整用 public float textSpacing; //行間の広さ public int fontSize; //フォントサイズ public float scrollHight = 25f; //スクロールする距離 /* msgSpeedは一文字を表示する間隔、lineSpeedは次の行を表示する間隔 */ public float msgSpeed = 0.05f, lineSpeed = 0.5f; private GameObject objManager, objMsgWindow, objMask; private GameObject[] objTextMsg = new GameObject[2]; private Transform trfCanvas; private RectTransform rText; private Text[] textMessage = new Text[2]; private string msgName; private string[] msg; private bool flg = false, flgStop = false, flgEnd = true, flgEom = true, flgScroll = false, flgDes = true; private int charCount = 1, mLength = 0, lineCount = 0; private float timer = 0f, scrollSpeed = 90f, scrollTimer = 0, textHight; Queue<string> qName = new Queue<string>(); Queue<string[]> qMsg = new Queue<string[]>(); Queue<bool> qDes = new Queue<bool>(); // Use this for initialization void Start () { trfCanvas = GameObject.Find("Canvas").transform; } /* メッセージが発生しているか否かを判別するための関数 */ public bool MessageEnd { get { return flgEom; } } public void CreateMsg(string mName, string[] message) { qName.Enqueue(mName); qMsg.Enqueue(message); qDes.Enqueue(true); } public void AddMsg(string mName, string[] message) { qName.Enqueue(mName); qMsg.Enqueue(message); qDes.Enqueue(false); } private void CreateWindow() { flgEom = false; string mName = qName.Dequeue(); msg = qMsg.Dequeue(); mLength = msg.Length; qDes.Dequeue(); if (mName != "system") { msgName = mName + "「"; } else { msgName = mName; } if (objMsgWindow == null) { objMsgWindow = (GameObject)Instantiate(preMessageWindow, Vector3.zero, Quaternion.identity, trfCanvas); objMsgWindow.transform.localPosition = windowPosition; RectTransform rectMsgWindow = objMsgWindow.GetComponent<recttransform>(); rectMsgWindow.sizeDelta = windowSize; objMsgWindow.transform.localScale = new Vector3(1f, 1f, 1f); objMask = (GameObject)Instantiate(preMask, Vector3.zero, Quaternion.identity, objMsgWindow.transform); objMask.transform.localPosition = textOffset; RectTransform rectMaskWindow = objMask.GetComponent<recttransform>(); rectMaskWindow.sizeDelta = textAreaSize; objMask.transform.localScale = new Vector3(1.0f, 1.0f, 1.0f); objTextMsg[0] = (GameObject)Instantiate(preText, Vector3.zero, Quaternion.identity, objMask.transform); objTextMsg[0].transform.localPosition = new Vector3(textOffset.x, textOffset.y - rectMaskWindow.sizeDelta.y / 2f, 0f); objTextMsg[0].transform.localScale = new Vector3(1f, 1f, 1f); rText = objTextMsg[0].GetComponent<recttransform>(); rText.sizeDelta = textAreaSize; textHight = textAreaSize.y; rText.pivot = new Vector2(0.5f, 0); textMessage[0] = objTextMsg[0].GetComponent<text>(); textMessage[0].text = ""; textMessage[0].fontSize = fontSize; textMessage[0].lineSpacing = textSpacing; objTextMsg[1] = (GameObject)Instantiate(preText, Vector3.zero, Quaternion.identity, objMsgWindow.transform); objTextMsg[1].transform.localPosition = new Vector3(0f, -windowSize.y / 2 + fontSize + 2, 0f); objTextMsg[1].transform.localScale = new Vector3(1f, 1f, 1f); textMessage[1] = objTextMsg[1].GetComponent<text>(); textMessage[1].text = ""; textMessage[1].alignment = TextAnchor.LowerCenter; textMessage[1].fontSize = fontSize - 4; if (msgName != "system") textMessage[0].text = msgName; } else { textMessage[0].text += "\n"; if (msgName != "system") textMessage[0].text += msgName; } flg = true; flgEnd = false; } /* 会話表示 */ void WriteMessage() { timer += Time.deltaTime; int nextLine = (lineCount + 1) % lineNumber; if (!flgStop && timer > msgSpeed) { if (lineCount != mLength) { if (charCount <= msg[lineCount].Length) { textMessage[0].text = textMessage[0].text + msg[lineCount].Substring(charCount - 1, 1); charCount += 1; timer = 0; if (preSndMessage != null) Instantiate(preSndMessage, Vector3.zero, Quaternion.identity); if (charCount == 2 && (lineCount > 2 || !flgDes)) { textHight = rText.sizeDelta.y; if (lineCount < mLength) flgScroll = true; if (nextLine == 1 && lineCount == mLength - 1) scrollHight *= 3f; if (nextLine == 2 && lineCount == mLength - 1) scrollHight *= 2f; } } else if (nextLine != 0) { charCount = 1; lineCount += 1; timer = -1f * lineSpeed; textMessage[0].text += "\n "; if (nextLine == 1 && lineCount == mLength) textMessage[0].text += "\n"; } else if (nextLine == 0 && charCount > msg[lineCount].Length) { lineCount += 1; if (lineCount != mLength) { textMessage[0].text += "\n"; textMessage[1].text = "▼"; flgStop = true; } } } } if (flgScroll == true) { scrollTimer += Time.deltaTime; if (rText.sizeDelta.y < textHight + scrollHight) { rText.sizeDelta = new Vector2(rText.sizeDelta.x, rText.sizeDelta.y + scrollSpeed * Time.deltaTime); } else { rText.sizeDelta = new Vector2(rText.sizeDelta.x, textHight + scrollHight); flgScroll = false; } } else { if (lineCount == mLength) flg = false; } } /* システムメッセージ */ void SystemMessage() { timer += Time.deltaTime; int nextLine = (lineCount + 1) % lineNumber; if (!flgStop && timer > lineSpeed) { if (lineCount < mLength - 1) { if (nextLine != 0) { if (lineCount > 2 || !flgDes) { textHight = rText.sizeDelta.y; flgScroll = true; if (nextLine == 1 && lineCount == mLength - 1) scrollHight *= 3f; if (nextLine == 2 && lineCount == mLength - 1) scrollHight *= 2f; } textMessage[0].text = textMessage[0].text + msg[lineCount] + "\n"; lineCount += 1; } else { if (lineCount != mLength - 1) { if (lineCount > 2 || !flgDes) { textHight = rText.sizeDelta.y; scrollHight = 25f; flgScroll = true; } textMessage[0].text = textMessage[0].text + msg[lineCount] + "\n"; textMessage[1].text = "▼"; lineCount += 1; flgStop = true; } } timer = 0; } else if(lineCount== mLength - 1) { if (lineCount > 2) { textHight = rText.sizeDelta.y; flgScroll = true; if (nextLine == 1) scrollHight *= 3f; if (nextLine == 2) scrollHight *= 2f; } textMessage[0].text = textMessage[0].text + msg[lineCount]; if (nextLine == 1) textMessage[0].text += "\n\n"; if (nextLine == 2) textMessage[0].text += "\n"; lineCount += 1; } } if (flgScroll == true) { scrollTimer += Time.deltaTime; if (rText.sizeDelta.y < textHight + scrollHight) { rText.sizeDelta = new Vector2(rText.sizeDelta.x, rText.sizeDelta.y + scrollSpeed * Time.deltaTime); } else { rText.sizeDelta = new Vector2(rText.sizeDelta.x, textHight + scrollHight); flgScroll = false; } } else { if (lineCount == mLength) flg = false; } } private void Main() { if (!flg && flgEnd && qName.Count > 0) { CreateWindow(); } if (flg == true) { if (msgName != "system") { WriteMessage(); } else { SystemMessage(); } if (Input.GetKeyDown(KeyCode.Space) && flgStop) { timer = -0.2f; charCount = 1; if (msgName != "system") textMessage[0].text += msgName; textMessage[1].text = ""; flgStop = false; textHight = rText.sizeDelta.y; scrollTimer = 0; if (preSndNextMessage != null) Instantiate(preSndNextMessage, Vector3.zero, Quaternion.identity); } } else if (flg == false) { if (Input.GetKeyDown(KeyCode.Space) && objMsgWindow != null) { charCount = 1; lineCount = 0; timer = 0; scrollHight = 25f; flgScroll = false; flgEnd = true; if(qName.Count == 0) flgEom = true; if (preSndNextMessage != null) Instantiate(preSndNextMessage, Vector3.zero, Quaternion.identity); if(qDes.Count > 0) flgDes = qDes.Peek(); if (flgDes || qName.Count == 0) { Destroy(objMsgWindow); Destroy(objTextMsg[0]); Destroy(objTextMsg[1]); } } } } // Update is called once per frame void Update() { Main(); } } </text></text></recttransform></recttransform></recttransform></bool></bool></string[]></string[]></string></string> |
使い方
先ほどのScriptを適当なゲームオブジェクトにドラック&ドロップでアタッチします。ゲームオブジェクトの名前をCreateMessageに変更します。
preMessageWindowへCreate→UI→Imageで作成し、メッセージウィンドウ用のゲームオブジェクトを、preTextにはCreate→UI→Textで作成したゲームオブジェクトprefabeにし、ドラック&ドロップで指定します。これらを。さらに、preSndMessageには一文字表示した際に再生する効果音を、preSndNextMessageには次のメッセージを表示する際に再生する効果音のprefabを指定します。Create→Audio→Audio Soruceから作成できます。
※効果音はなくても問題ありません。
ここまでは前回のScriptと同様です。
preMaskにCreate→UI→Imageを作成し、ColorのAlphaを0にします。このImageにAddComponentからRectMask2Dをアタッチします。このゲームオブジェクトをprefabにし、preMaskに指定します。ScrollHightはメッセージをスクロールする距離です。フォントサイズや行間によって変わるので、適当な数値を入れてください。
次に新しく下記のようなScriptを作成します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
using System.Collections; using System.Collections.Generic; using UnityEngine; public class MessageTest : MonoBehaviour { private CreateMessage createMessage; private bool flg = true; // Use this for initialization void Start () { GameObject obj; obj = GameObject.Find("CreateMessage"); if (obj != null) createMessage = obj.GetComponent<CreateMessage>(); } // Update is called once per frame void Update () { if (flg) { string[] msg = { "テストメッセージ", "テストメッセージ", "テストメッセージ", "テストメッセージ", "テストメッセージ" }; createMessage.CreateMsg("あああ", msg); createMessageV5.AddMsg("いいい", msg); createMessage.CreateMsg("system", msg); flg = false; } if (createMessage.MessageEnd) { Debug.Log("メッセージは表示されていません。"); } else { Debug.Log("メッセージは表示されています。"); } } } |
createMessage.CreateMsg(名前, 表示するメッセージ)でメッセージを表示することができます。通常は一文字ずつ表示されますが、名前の部分にsystemと入力すると、一行ずつ表示されるようになります。また、表示したいメッセージはString型の配列を指定します。createMessage.AddMsg(名前, 表示するメッセージ)で前回のメッセージを削除せずに、続きから新しいメッセージを表示することができます。また、createMessage.MessageEndでメッセージが表示されているか否かを判別できます。
新しくゲームオブジェクトを作成し、このScriptをアタッチし、実行するとメッセージが表示されます。
バグ等、何かありましたらコメントもしくはお問い合わせいただけると幸いです。
-
前の記事
メッセージを表示するScript(2018/7/21修正) 2018.07.15
-
次の記事
rigidbodyの軌道予測線を作成してみた(空気抵抗なし) 2018.08.01
コメントを書く